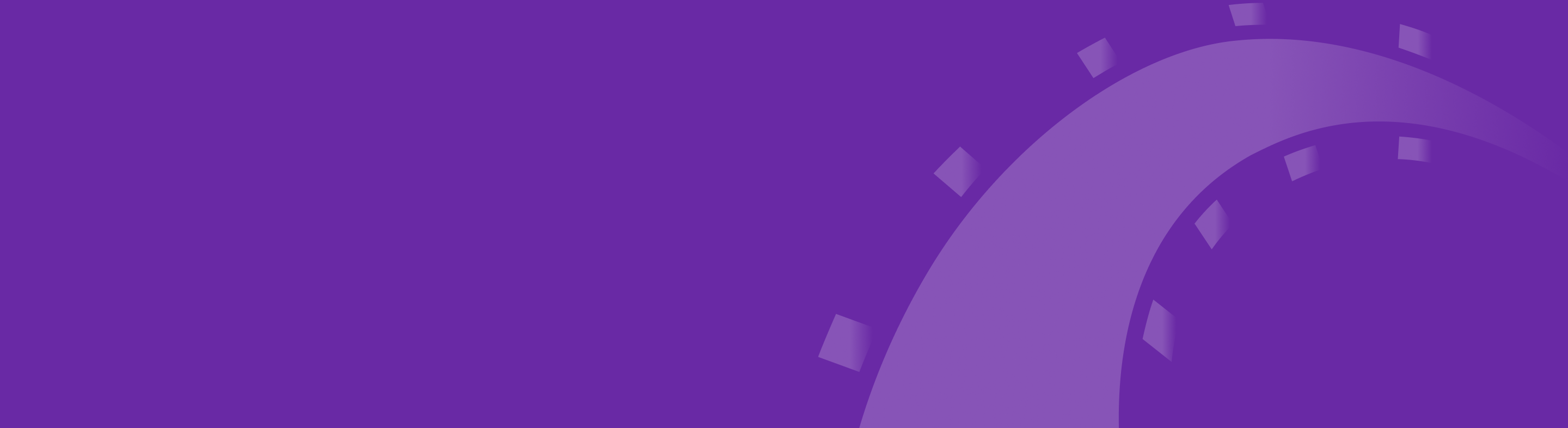
Announcing Ruby on Rails native support for Outropy!
Outropy is the AI platform for product engineers
Build AI features like modern software—with clean code, not research papers. Break problems into small, reusable tasks that are easy to test, maintain, and deploy.
Deliver AI that is reliable, scalable, and actually useful.
Task-Oriented AI,
Built for Developers
Break your AI system into tasks—clean, single-purpose functions that compose like regular code. Each task understands your data model and accesses what it needs with minimal setup.Build sophisticated AI by composing tasks while keeping your code clear, modular, and maintainable.
1// Available roles for candidates
2const possibleRoles: Role[] = [
3 { name: 'Junior Software Engineer' },
4 { name: 'Senior Software Engineer' },
5 { name: 'Engineering Manager' },
6 { name: 'Data Scientist' },
7 { name: 'SRE' },
8];
9
10export async function generateCandidateLeadForHiringCompany(resumes: Resume[]): Promise<void> {
11 const rolesForCandidateClassifier = await outropy.createClassifyTask({
12 name: 'find_roles_for_candidate',
13 instructions: 'From this resume, find the roles that the candidate is qualified for',
14 guidelines: [
15 'Use only the described experience and skills of the candidate',
16 'Do not take into account the location or the name of the candidate',
17 ],
18 options: possibleRoles,
19 });
20
21 const generateDossier = await outropy.createExplainerTask({
22 name: 'generate_dossier',
23 instructions: 'Generate a dossier explaining why the candidate is a good fit for the role',
24 guidelines: [
25 'Focus on the individual experiences and skills of the candidate',
26 'Do not take into account the location or the name of the candidate',
27 ],
28 });
29
30 const summarizeDossiers = await outropy.createContextualizerTask({
31 name: 'summarize_dossiers',
32 instructions: 'From this list of dossiers, generate a summary for the hiring company',
33 guidelines: [
34 'Give a brief overview of the candidate's experience and skills',
35 'Do not add the name of the candidate in the summary',
36 'Highlight experiences at companies similar to the hiring company',
37 ],
38 });
39
40 const candidatesByRole = new Map<Role, Resume[]>(
41 possibleRoles.map((role) => [role, []])
42 );
43
44 for (const resume of resumes) {
45 // Utilizes Outropy to classify the roles for the candidate
46 const roles = await outropy.executeTask<Role[]>(rolesForCandidateClassifier, resume);
47
48 for (const role of roles) {
49 const candidates = candidatesByRole.get(role) ?? [];
50
51 candidates.push(resume);
52 candidatesByRole.set(role, candidates);
53 }
54 }
55
56 // Process each role and company
57 for (const role of possibleRoles) {
58 const candidatesForThisRole = candidatesByRole.get(role) ?? [];
59 const companiesHiringForThisRole = hiringCompaniesDatabase.findAllByRole(role);
60
61 for (const hiringCompany of companiesHiringForThisRole) {
62 const dossiersForCandidates = await Promise.all(
63 // Utilizes Outropy to generate a dossier with detailed info about why the candidate is a good fit for the company
64 candidatesForThisRole.map(async (candidate) =>
65 outropy.executeTask<string>(generateDossier, { candidate, role })
66 )
67 );
68
69 // Utilizes Outropy to summarize all dossiers for the hiring company into a single email
70 const summaryOfAllDossiersForThisCompany = await outropy.executeTask<string>(
71 summarizeDossiers,
72 { hiringCompany, dossiers: dossiersForCandidates },
73 );
74
75 await sendEmail(hiringCompany.contact, summaryOfAllDossiersForThisCompany);
76 }
77 }
78}
Automate Away the Trial-and-Error, Focus on Building
Most AI projects waste time on trial-and-error instead of delivering real value.
Outropy automates experimentation, analysis, and optimization—so you can focus on shipping great products.
Experimentation
Outropy runs automated experiments on models, hyperparameters, and preprocessing techniques to find the best combination for your data—like A/B testing, but for AI.
Continuous Analysis
Even small changes in data or models can affect AI performance. Outropy detects shifts and adapts pipelines automatically, ensuring consistent, reliable results.
Visibility & Observability
Stay in control with real-time monitoring via UI or API. Outropy also integrates with your existing observability, alerting, and FinOps tools.
Your apps with
state-of-the-art AI
Constantly figuring out which model performs best for each task is a distraction from building great software. Outropy automates model selection, choosing the optimal model for each task in real time. Focus on building features, not benchmarking.
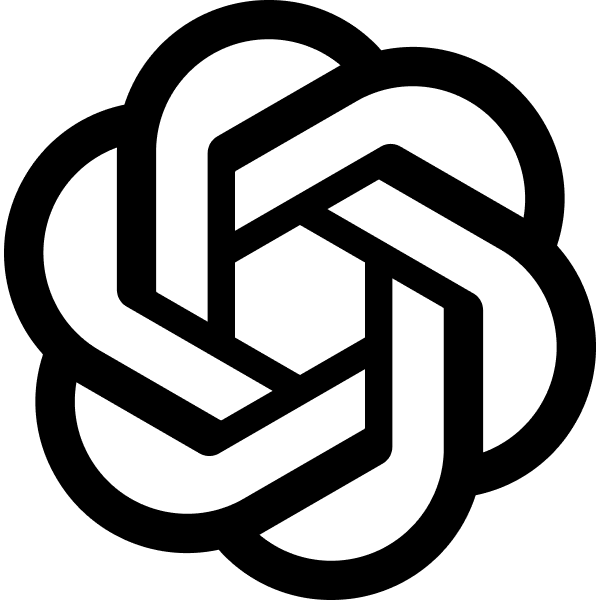
OpenAI
Use OpenAI's GPT-4o, GPT-3.5, and the latest ChatGPT models.
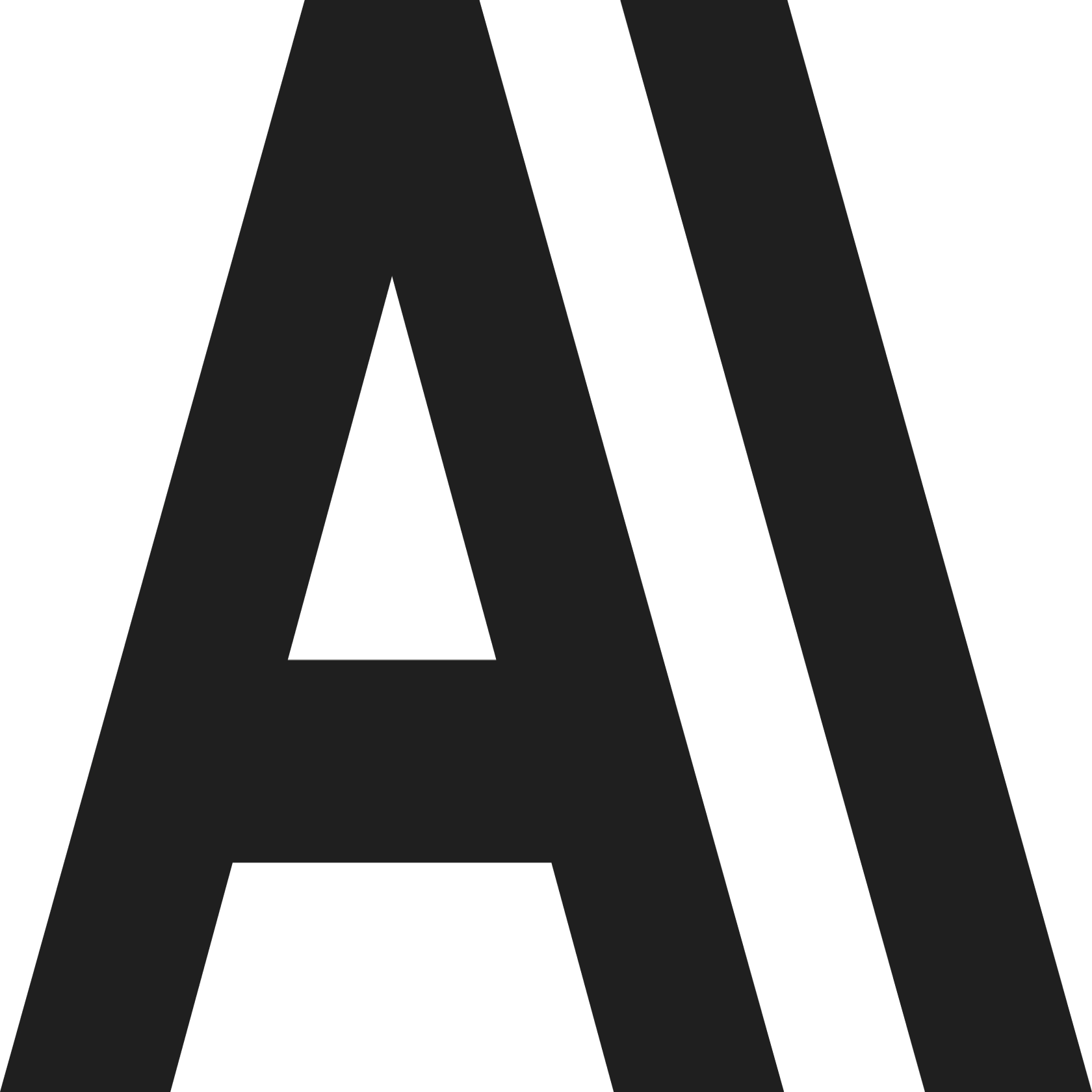
Anthropic
Leverage Claude's best-in-class models for computer vision and natural language.
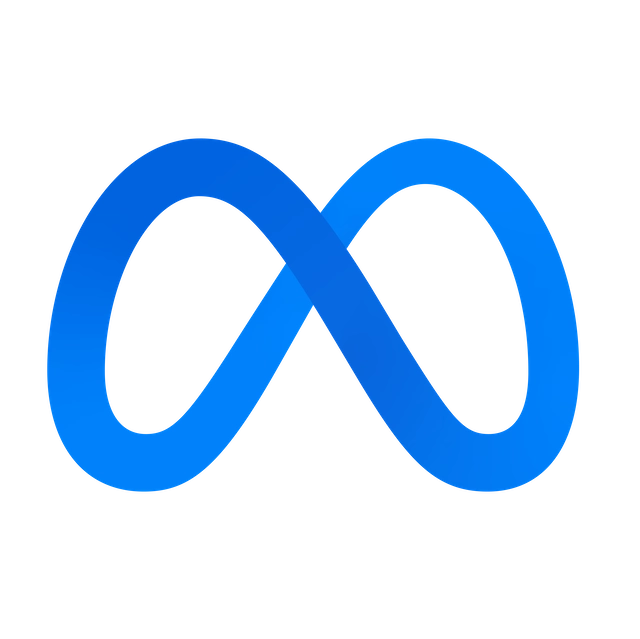
Meta's Llama 3.1
The most advanced open-source AI models, running on the cloud or on-premises.
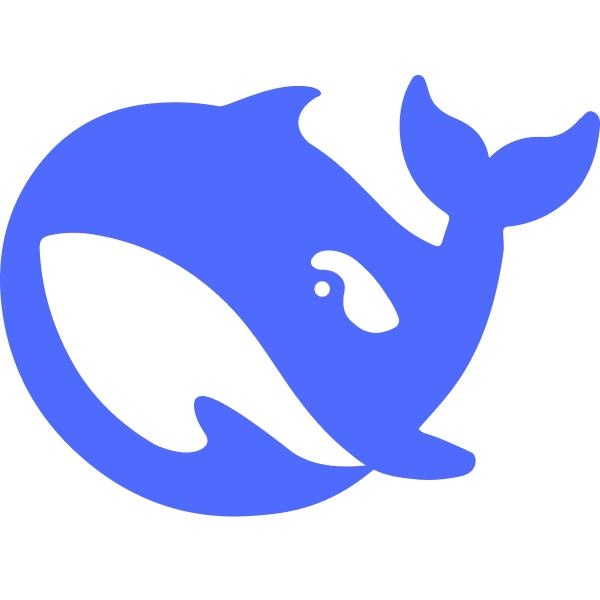
DeepSeek
DeepSeek combines powerful AI performance with surprisingly low costs.
SDKs for many languages,
API for the REST
Outropy SDKs are designed to provide a native experience in your favorite programming language and framework.
1async function main(): Promise<void> {
2 const generateProjectUpdateTask = await outropy.createTask({
3 taskType: TaskTypes.GENERATE,
4 taskName: "generate_project_update",
5 inputType: StandupMeetingTranscript,
6 outputType: ProjectUpdatePost,
7 instructions: "Generate a post with project updates based on the standup meeting transcript.",
8 guidelines: [
9 "Include project updates, blockers, and action items.",
10 "Do not include personal updates or banter."
11 ],
12 referenceData: [projectDatabase, employeeDatabase],
13 examples: [
14 [SAMPLE_TRANSCRIPT1, SAMPLE_POST1],
15 [SAMPLE_TRANSCRIPT2, SAMPLE_POST2]
16 ],
17 directives: new Directives({ latency: 0.1, cost: 0.5, accuracy: 0.4 })
18 });
19
20 const projectUpdate = await outropy.executeTask({
21 taskId: generateProjectUpdateTask,
22 subject: standupTranscript
23 });
24
25 await userFeeds.post(projectUpdate);
26}
AI, for Developers
Skip the trial-and-error and start building powerful AI features—clean, composable, and production-ready.